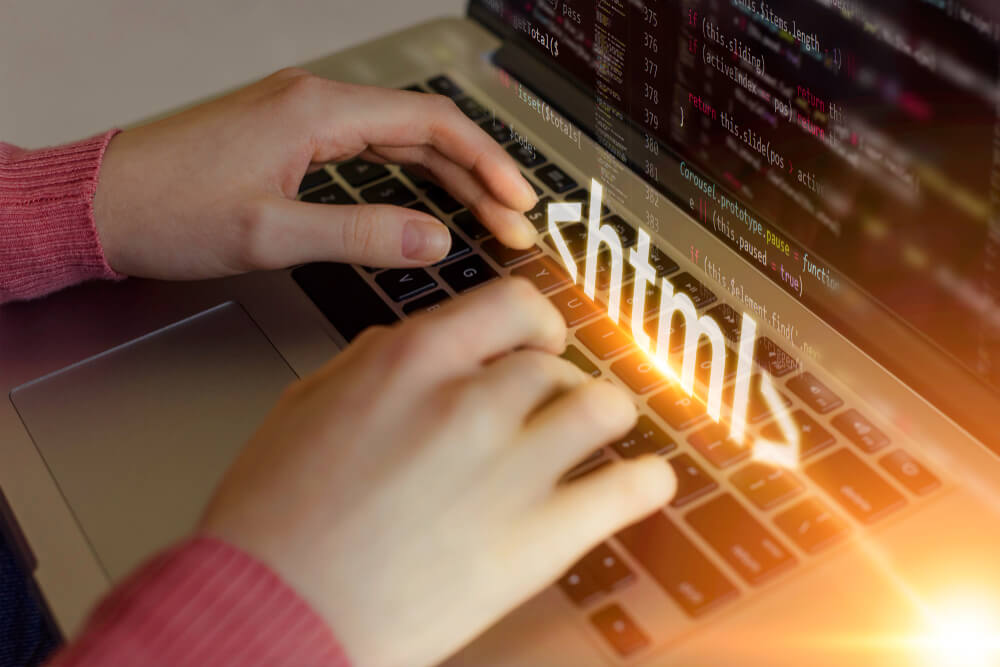
What is Web Scraping?
Web scraping involves collecting information from websites. This data is then saved to a local file or database for further analysis. Essentially, web scraping involves fetching the underlying HTML code of a webpage and sifting through it to find and retrieve the specific data you’re interested in. This technique is incredibly useful for gathering large amounts of data from the web automatically, saving time and effort compared to manual data collection.
In more technical terms, web scraping involves making HTTP requests to web servers, parsing the HTML response, and extracting relevant information using various programming techniques. The scraped data can be in different formats such as text, images, or even entire web pages. The process can be fully automated with scripts and software, enabling efficient data collection from multiple sources simultaneously.
Types of Data You Can Scrape
The types of data you can scrape are vast and varied. Here are some common examples:
- Text Content: Extracting articles, blog posts, and reviews.
- Images: Collecting images for analysis or storage.
- Product Data: Gathering information such as prices, descriptions, and reviews from e-commerce sites.
- Contact Information: Retrieving emails, phone numbers, and addresses from directories.
- Social Media Data: Collecting posts, comments, likes, and various other social media interactions.
- Financial Data: Collecting stock prices, market data, and financial reports.
- Weather Data: Gathering historical and real-time weather information.
- Job Listings: Extracting job postings and associated details.
- Government Data: Collecting public records and reports.
By scraping these types of data, businesses, researchers, and developers can gain valuable insights, build datasets for machine learning, and enhance their applications with real-time information. This process allows for the aggregation of diverse data sources, providing a comprehensive view of trends, user behavior, and market dynamics. For instance, businesses can analyze customer reviews to improve their products, researchers can compile data for more accurate studies, and developers can integrate up-to-date information into their apps. If you’re looking to leverage these capabilities effectively, it’s crucial to hire vetted developers who are skilled in web scraping techniques and data analysis.
Applications of Web Scraping
Web scraping has a multitude of applications across various fields. Here are some common uses:
- Market Research: Collecting data on competitors’ products and pricing to gain market insights and identify trends.
- Academic Research: Gathering large datasets for analysis in studies and experiments.
- News Aggregation: Compiling news articles from different sources for a centralized feed.
- Price Comparison: Extracting product prices from multiple sites to find the best deals for consumers.
- Sentiment Analysis: Analyzing customer reviews and feedback to gauge public opinion on products or services.
- Lead Generation: Extracting potential customer contact information for sales and marketing purposes.
- Content Aggregation: Collecting and curating content from various sources for websites or newsletters.
- Data Journalism: Gathering data for investigative journalism and data-driven stories.
- SEO Monitoring: Tracking website rankings and keyword performance.
- Real Estate Analysis: Collecting property listings and market data for real estate analysis.
By automating data collection, web scraping enables organizations to make data-driven decisions, enhance their services, and stay competitive in their respective industries.
The Functions of Web Scraping
A web scraper functions through a series of steps designed to fetch and process data from a website. Here’s how it works:
Step 1: Making an HTTP Request
The first step involves sending an HTTP request to the server hosting the target website. This request asks the server to send back the HTML content of the page. Commonly, tools like requests in Python are used to handle this process efficiently.
When making an HTTP request, it’s essential to handle different types of requests (GET, POST, etc.) based on the website’s requirements. A GET request retrieves data from the server, while a POST request submits data to be processed. Properly structuring these requests ensures successful communication with the web server.
Step 2: Extracting and Parsing Website Code
Once the HTML content is retrieved, the next step is to parse this code to extract the relevant data. This involves identifying and isolating specific elements within the HTML, such as tags containing the desired information. Libraries like BeautifulSoup or Scrapy in Python are often used for parsing HTML.
Parsing involves traversing the HTML tree structure and finding elements using selectors (e.g., CSS selectors or XPath). It’s important to understand the structure of the HTML document to accurately locate the required data. Advanced parsing techniques may involve handling JavaScript-rendered content using tools like Selenium or Puppeteer.
Related read: Avoiding Parsing Errors When Web Scraping
Step 3: Saving Data Locally
After extracting the data, it should be saved in a useful format such as a CSV file, JSON file, or a database. The suitable format will vary based on how the data is intended to be used.
Efficient data storage requires choosing the right storage method and format. For example:
- CSV Files: Suitable for tabular data and easy integration with spreadsheet applications.
- JSON Files: Ideal for hierarchical or nested data structures.
- Databases: Useful for large datasets requiring complex queries and relationships.
By saving the data in the right format, you can ensure easy access, analysis, and integration with other systems.
Step-by-Step Guide to Web Scraping
Step 1: Find the URLs You Want to Scrape
Locate the web pages that contain the necessary data. List these URLs, ensuring they are accessible and contain the desired information.
Step 2: Inspect the Page
Use your web browser’s developer tools (usually accessible by right-clicking on the page and selecting “Inspect”) to examine the HTML structure of the target page. This will help you locate the specific elements you need to extract.
When inspecting the page, look for:
- HTML Tags: Identify the tags containing your target data (e.g., <div>, <span>, <a>).
- Classes and IDs: Use class and ID attributes to narrow down specific elements.
- Data Attributes: Some websites use custom data attributes (e.g., data-price) to store relevant information.
Step 3: Identify the Data You Want to Extract
Pinpoint the exact HTML tags and attributes where your data resides. For example, if you’re scraping product prices, you might look for tags like <span class=”price”>.
Step 4: Write the Necessary Code
Write a script to automate the scraping process. Here’s a basic Python example utilizing BeautifulSoup:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find_all('span', class_='price')
for item in data:
print(item.text)
In this example, the requests library is used to fetch the HTML content of the page, and BeautifulSoup is used to parse the HTML and extract data.
Step 5: Execute the Code
Run your script to start scraping data. Ensure that your code handles exceptions and errors gracefully, such as timeouts or unexpected changes in the webpage structure.
Step 6: Storing the Data
Save the scraped data to your preferred format. For example, you could write the data to a CSV file:
import csv
with open('data.csv', mode='w') as file:
writer = csv.writer(file)
writer.writerow(['Price'])
for item in data:
writer.writerow([item.text])
By following these steps, you can effectively scrape and store data from websites, enabling further analysis and use.
Tools for Web Scraping
There are various tools available for web scraping, each with its own strengths and use cases. Here are some popular ones:
1. ParseHub
ParseHub is a powerful web scraping tool that can handle complex scraping tasks without requiring extensive programming knowledge. It offers a visual interface for defining the data extraction process and supports JavaScript-heavy websites.
Key Features:
- Visual Interface: Allows users to click and point to the data they want to extract.
- API Support: Provides an API for integrating scraped data with other applications.
- Handles Dynamic Content: Can scrape content generated by JavaScript.
2. Pandas
Pandas is a Python library widely used for data manipulation and analysis. While not a scraping tool per se, it’s incredibly useful for processing and cleaning scraped data. You can combine it with libraries like BeautifulSoup for an end-to-end scraping and data analysis solution.
Key Features:
- DataFrames: Provides powerful DataFrame structures for handling tabular data.
- Integration: Works seamlessly with other Python libraries like NumPy and Matplotlib.
- Data Cleaning: Offers extensive functions for cleaning and transforming data.
3. Scrapy
Scrapy is a robust Python framework specifically designed for web scraping. It provides built-in support for handling requests, parsing HTML, and exporting data in various formats. Scrapy is suitable for more advanced scraping tasks and projects requiring high performance.
Key Features:
- Crawling Capabilities: Can handle large-scale scraping projects with ease.
- Customizability: Allows for custom spiders and middleware for specific scraping needs.
- Data Export: Supports exporting data in formats like CSV, JSON, and XML.
4. BeautifulSoup
BeautifulSoup is a popular Python library that is commonly used for handling and parsing HTML and XML documents. It provides a simple and intuitive interface for navigating and searching the HTML tree, making it ideal for beginners. BeautifulSoup is often used in combination with the requests library for fetching web page content.
Key Features:
- Easy to Use: Simple API for beginners to quickly learn and use.
- Flexible Parsing: Can parse HTML and XML from various sources.
- Integration: Works well with other libraries like requests and lxml.
Ethical Considerations and Best Practices
While web scraping can be incredibly useful, it’s important to adhere to ethical guidelines and best practices:
1. Respect Robots.txt
Many websites have a robots.txt file that specifies which parts of the site can be accessed by web crawlers. Always ensure to check and adhere to these guidelines to avoid scraping prohibited sections of the site.
2. Avoid Overloading Servers
Sending too many requests in a short period can overload a server, causing it to crash or block your IP address. Implement rate limiting and pauses between requests to prevent this.
3. Attribute Sources
If you’re using scraped data publicly, always attribute the source of the data. This not only acknowledges the original creator but also builds trust with your audience.
4. Stay Compliant
Ensure that your web scraping activities comply with legal requirements, such as the General Data Protection Regulation (GDPR) in Europe or other relevant laws in your jurisdiction.
Advanced Web Scraping Techniques
As you get more adept at basic web scraping, you may face more intricate situations that demand advanced methods.
1. Handling JavaScript-Rendered Content
Some websites use JavaScript to load content dynamically. Traditional scraping methods may not work, as the data isn’t present in the initial HTML response. Tools like Selenium or Puppeteer can be used to render JavaScript content and scrape it effectively.
2. Using APIs
Some websites provide APIs for accessing data programmatically. Using an API can be more efficient and reliable than scraping, as it provides structured data in a predictable format. Always verify the availability of an API before opting for web scraping.
3. Managing Authentication
Certain websites require authentication to access data. In these cases, you may need to handle login sessions and cookies. Libraries like requests in Python support session management, allowing you to maintain authentication while scraping.
4. Distributed Scraping
For large-scale scraping projects, distributing the load across multiple machines can improve efficiency and prevent IP blocking. Distributed frameworks like Scrapy Cluster or Apache Nutch can help manage this process.
Common Challenges in Web Scraping
Web scraping isn’t always straightforward. Here are common challenges you might face and methods to address them effectively:
1. Changing Website Structure
Websites can change their structure without notice, breaking your scraping scripts. Regularly monitor and update your scripts to handle these changes.
2. Captchas
To prevent automated access, some websites use captchas. Solving captchas programmatically can be challenging. Services like 2Captcha or Anti-Captcha can help, but it’s best to look for alternative ways to access the data.
3. IP Blocking
Websites might restrict your IP address if they notice an excessive amount of scraping activity. Using proxies and rotating IP addresses can help avoid this issue. Services like ScraperAPI or Bright Data offer proxy solutions for web scraping.
Real-World Examples of Web Scraping Projects
1. E-Commerce Price Monitoring
An online retailer can use web scraping to monitor competitors’ prices and adjust their prices accordingly. This ensures they remain competitive in the market and can attract more customers with the best deals.
2. Real Estate Listings Aggregation
A real estate agency can scrape listings from various websites to provide a comprehensive view of the market. This helps clients find properties more easily and gives the agency a competitive edge.
3. Sentiment Analysis of Product Reviews
A company can scrape product reviews from multiple sources to analyze customer sentiment. This information can guide product development, enhance marketing strategies, and improve customer service.
4. Financial Data Collection
Investors and analysts can scrape financial reports, stock prices, and market data to make informed investment decisions. This provides a competitive advantage by having access to real-time data.
In Summary
Web scraping is a powerful technique for automating data extraction from websites. Whether you’re conducting market research, gathering academic data, or simply compiling information for personal use, web scraping can save you time and effort. By understanding the basics and utilizing the right tools, you can efficiently scrape and analyze web data.
Remember to always respect the terms of service of the websites you scrape and to use scraping responsibly. Happy scraping!
With this comprehensive guide, you now know how to start your web scraping journey. Whether you’re a beginner looking to extract data for a personal project or a professional aiming to gather large datasets, web scraping offers a versatile solution to meet your needs. By following best practices and using the right tools, you can unlock the full potential of web scraping and harness valuable data from the vast expanse of the internet.