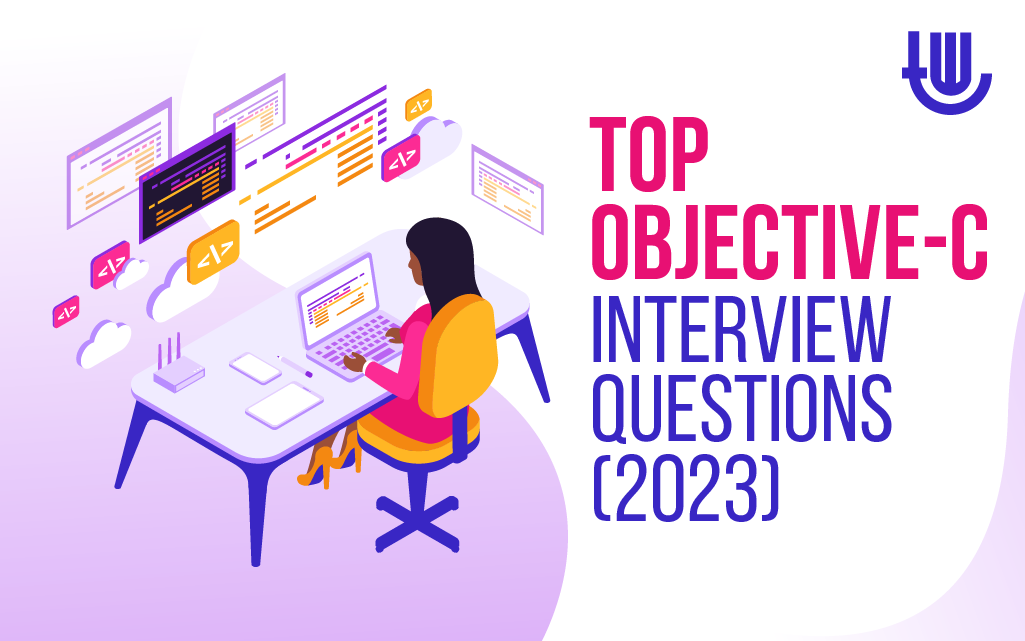
Objective-C is an object-oriented programming language developers use to create applications and software for Apple products.
It’s a powerful language, but it’s also complex and challenging to learn – as such, there are always plenty of questions about it in job interviews.
If you’re looking for a job with Objective-C coding requirements or want to brush up on the language before an interview, this blog post is for you.
We have compiled a list of some of the most common Objective-C questions asked during job interviews in 2023, so you can be prepared for any possible curve balls thrown your way.
What is Objective-C?
Objective-C is a powerful, object-oriented programming language for building sophisticated software applications. It was created in the early 1980s by Brad Cox and Tom Love.
Objective-C is based on the C programming language but adds several important features that make it more powerful and flexible.
One of the most critical features of Objective-C is its support for object-oriented programming (OOP). OOP is a way of organizing code that focuses on creating reusable objects.
This can make code easier to write and maintain. Objective-C also has strong support for Dynamic Runtime, allowing developers to add new functionality to existing classes at runtime dynamically.
In addition to its robust feature set, Objective-C is easy to learn. It has a simple syntax that is easy to read and understand. And because it builds on the C programming language, many programmers already have some familiarity.
What are the main features of Objective-C?
Objective-C is a general-purpose, object-oriented programming language that adds Smalltalk-style messaging to the C programming language.
It was the primary programming language used by Apple for the OS X and iOS operating systems and their respective application programming interfaces (APIs), Cocoa and Cocoa Touch, before the introduction of Swift.
What is an interface in Objective-C?
An interface in Objective-C is a formal protocol that defines a set of methods a class must implement.
An interface can be @protocol (in which case it cannot be used to define instances of classes) or an NSObject subclass (in which case it can be used to define instances of classes).
What is a category in Objective-C?
In Objective-C, a category is a named group of related methods that can be added to an existing class. Categories are often used to add functionality to existing classes without subclassing them.
Categories can be used to:
- Add new methods to an existing class
- Override existing methods
- Declare new properties
- Define class behavior
How do you define a protocol in Objective-C?
In Objective-C, a protocol is an interface that defines a set of methods that any class can implement. Protocols are similar to Java interfaces but can also include optional methods and properties.
Protocols can be used to define delegate protocols, which specify the methods that a delegate object must implement.
What are delegates in Objective-C?
In Objective-C, delegates represent objects that can perform specific tasks on behalf of other objects. Delegates can be used for various purposes, such as handling events, providing data to a table view, or implementing protocols.
How do you create a singleton class in Objective-C?
To create a singleton class in Objective-C, you need to follow these three steps:
- Define a static instance variable for the class.
- Write a custom initializer method that initializes the instance variable and sets it to nil.
- Implement a class method that returns the static instance variable.
Here is an example of how to do this:
static MyClass *sharedInstance = nil;
+ (MyClass *)sharedInstance { if (sharedInstance == nil) { sharedInstance = [[super allocWithZone:NULL] init]; } return sharedInstance;}- (id)init { if (self = [super init]) { // Initialize here... } return self;}
Conclusion:
In conclusion, Objective-C remains a popular programming language for developing apps for various platforms, and preparing yourself with the most commonly asked interview questions can help increase your chances of landing your desired job.
It’s essential to review these questions, whether you’re a seasoned developer or just starting out, to get an idea of what to expect and effectively showcase your skills and knowledge.
Familiarizing yourself with these questions is a smart investment in your career and a great way to stay ahead of the game in 2023 and beyond.